This is great option to use when you’re unit testing a web-based application or need to do testing that revolves around using a Web browser.
Selenium is an open-source automation tool used in this situation — https://www.seleniumhq.org/ . You can use Selenium with pretty much any language you want, but in this example, I’m choosing to use Python 3.
Very easy guide can be found at https://pypi.org/project/selenium/ which will give you the basics on how to install Selenium via pip.
Once you have this installed, you’ll need to make sure you have WebDrivers for each browser you wish to use. In my case, I’m just using Firefox. It’s not explained too well how to use the driver, but essentially you just need to add the driver directory or copy the driver into a directory that is present in your environment path (my OS of choice is Fedora, so this will be the $PATH environment variable).
I’ve taken the example shown in the easy guide, and adjusted based on an excellent tutorial at https://www.internalpointers.com/post/run-painless-test-suites-python-unittest to show how Python’s unittest library and Selenium work together — I made two small Python files to handle the test:
browser_test.py
import unittest
from selenium import webdriver
class BrowserTestCase(unittest.TestCase):
def setUp(self):
self.browser = webdriver.Firefox()
self.addCleanup(self.browser.quit)
def testPageTitle(self):
self.browser.get('http://ittech.expert')
self.assertIn('Nathan', self.browser.title)
driver.py
import unittest
# Import our browser test case
import browser_test
# Init test suite
test_loader = unittest.TestLoader()
test_suite = unittest.TestSuite()
# Add tests to suite
test_suite.addTests(test_loader.loadTestsFromModule(browser_test))
# Run the test!
test_runner = unittest.TextTestRunner(verbosity=2)
test_result = test_runner.run(test_suite)
Testing it out…
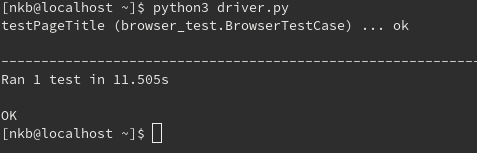